I recently completed the QuantNet Advanced C++ course and shared my testimonial here. I have several members expressing interest in how I apply specific modern techniques to my job. I hope this will spur more interest in the course and explain things that you can do to generate savings in latency, and running costs which in turn result in huge cost savings.
I work for a large advertising business on the auction side. We received a series of bids from the advertisers and have to show ads to help advertisers and creators while not being overly annoying to the users.
The business has giant scales with hundreds of billions of ad queries daily. The latency requirement is not quite as crazy as HFT, but ads still have to be served within 1 second, and within that 1 second, a lot of memory, CPU, and GPU are used. Because of the giant scale of the business, every tiny resource-saving of just milliseconds gets scaled by hundreds of billions
We have a system to calculate several statistics of the ad candidate relating to the history of other ads that the users have seen in the past (to avoid ads being repeated). A lot of these counts are inefficient for loops that were sufficient when the system did not include crazy ML models but are now a significant latency hog. I transformed all for loops into ranges-based and devised a thread-based scheme to calculate these stats in parallels (because these stats are independent across different candidates). Doing so helped reduce the latency, which saved several years of SWEs for the company and allowed more ms for another more resource-intensive project to launch.
There are a lot of other smaller savings as well. One time, I literally just changed a struct copy to a reference. Because that structure is so big and so popular, doing so ends up being a huge savings. Or the other time I changed the key from string concatenation to tuple. The savings are really easy to monitor because the company did an excellent job tracking resource usage, down to every individual function call. Now that I know all these excellent C++ features, I expect many more savings in the future.
I hope the examples above illustrate the numerous ways one can leverage the modern C++ technique to improve legacy code in significant ways. Even outside of quant, learning C++ optimization techniques are still extremely useful, especially for large-scale systems that compute a lot of small tasks.
I'm happy to answer any questions you have about these features or other parts of my work.
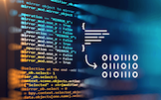
I work for a large advertising business on the auction side. We received a series of bids from the advertisers and have to show ads to help advertisers and creators while not being overly annoying to the users.
The business has giant scales with hundreds of billions of ad queries daily. The latency requirement is not quite as crazy as HFT, but ads still have to be served within 1 second, and within that 1 second, a lot of memory, CPU, and GPU are used. Because of the giant scale of the business, every tiny resource-saving of just milliseconds gets scaled by hundreds of billions
We have a system to calculate several statistics of the ad candidate relating to the history of other ads that the users have seen in the past (to avoid ads being repeated). A lot of these counts are inefficient for loops that were sufficient when the system did not include crazy ML models but are now a significant latency hog. I transformed all for loops into ranges-based and devised a thread-based scheme to calculate these stats in parallels (because these stats are independent across different candidates). Doing so helped reduce the latency, which saved several years of SWEs for the company and allowed more ms for another more resource-intensive project to launch.
There are a lot of other smaller savings as well. One time, I literally just changed a struct copy to a reference. Because that structure is so big and so popular, doing so ends up being a huge savings. Or the other time I changed the key from string concatenation to tuple. The savings are really easy to monitor because the company did an excellent job tracking resource usage, down to every individual function call. Now that I know all these excellent C++ features, I expect many more savings in the future.
I hope the examples above illustrate the numerous ways one can leverage the modern C++ technique to improve legacy code in significant ways. Even outside of quant, learning C++ optimization techniques are still extremely useful, especially for large-scale systems that compute a lot of small tasks.
I'm happy to answer any questions you have about these features or other parts of my work.
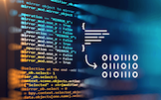
Last edited by a moderator: